Embedded Chat
Add a chat UI directly on a dedicated page
Overview
An "embedded chat" is a chat UI that is directly incorporated into a page instead of within a pop-up modal. The embedded chat UI looks like this:
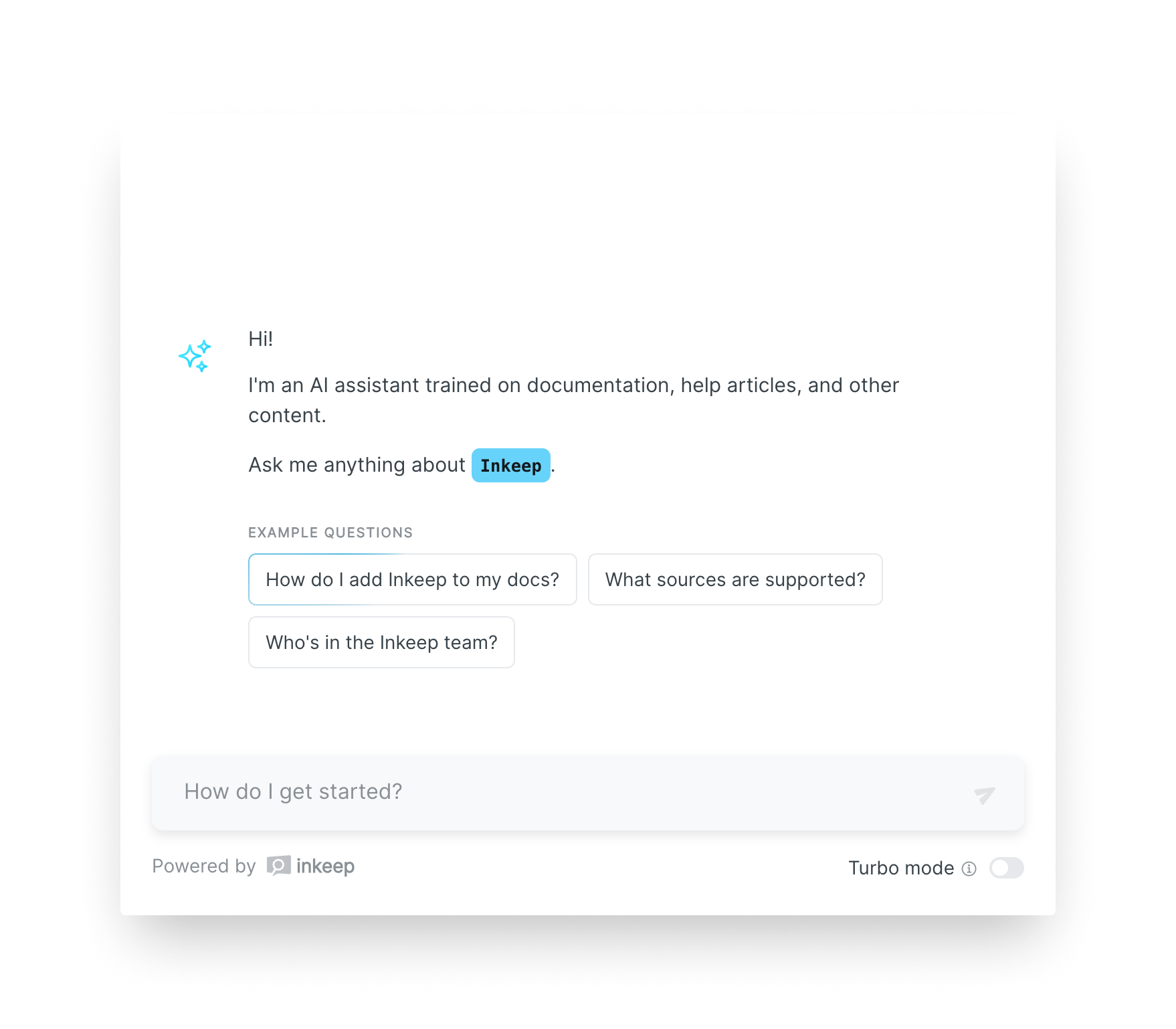
Scenarios
You may want to add an embedded chat in places where you want to encourage the user to interact with the AI chat directly. For example, an embedded chat can be useful to:
- Deflect questions in your help center site
- Provide an AI chat experience if your documentation site doesn't allow third party components, like for GitBook and Readme.
- Create a place for sharable chat sessions
You can incorporate the embedded chat directly on an existing page like help.domain.com
or create a dedicated one like domain.com/ask-ai
.
Quick start
Add the below <script>
tag to the <head>
or <body>
of your website.
<!-- Add Inkeep widget -->
<script
type="module"
src="https://unpkg.com/@inkeep/widgets-embed@0.2.278/dist/embed.js"
defer
></script>
Define an element in your page that will be the “container” for the embedded chat.
<div style="display: flex; align-items: center; justify-content: center; height: calc(100vh - 16px);">
<div style="max-height: 600px; height: 100%;">
<div id="ikp-embedded-chat-target"></div>
</div>
</div>
Insert the Embedded Chat by using the Inkeep.embed()
function.
<script type="module" defer>
const inkeepWidget = Inkeep().embed({
componentType: "EmbeddedChat", // required
targetElement: document.getElementById("ikp-embedded-chat-target"), // required
properties: {
stylesheetUrls: ['/path/to/stylesheets'], // optional
// optional -- for syncing UI color mode
colorModeSync: {
observedElement: document.documentElement,
isDarkModeCallback: (el) => {
return el.classList.contains("dark");
},
colorModeAttribute: 'class',
},
baseSettings: {
apiKey: process.env.INKEEP_INTEGRATION_API_KEY!, // required
integrationId: process.env.INKEEP_INTEGRATION_ID!, // required
organizationId: process.env.INKEEP_ORGANIZATION_ID!, // required
organizationDisplayName: "Inkeep",
primaryBrandColor: "#26D6FF",
//... optional base settings
},
modalSettings: {
// optional InkeepModalSettings
},
searchSettings: {
// optional InkeepSearchSettings
},
aiChatSettings: {
// optional InkeepAIChatSettings
botAvatarSrcUrl: "/img/inkeep-logo.svg", // use your own bot avatar
botAvatarDarkSrcUrl: "/img/inkeep-logo-dark.svg" // for dark mode or more contrast against button bg
quickQuestions: [
"Example question 1?",
"Example question 2?",
"Example question 3?",
],
}
},
});
</script>
Styling
Inkeep.Embed() for Embedded Chat
Parameter | Type | Description |
---|---|---|
componentType | string | Required. Must be EmbeddedChat to add the EmbeddedChat component. |
targetElement | HTMLElement | Required. A valid HTML element where you want to render the EmbeddedChat component. |
properties | InkeepEmbeddedChatProps | Required. An object that contains the base settings and other optional settings for the Embedded Chat |
colorModeSync | ColorModeSync | An object that contains the settings to sync the color mode in the widgets with the parent UI. |
ColorModeSync
These setting can be used to sync the widget's color mode with the color mode set in the parent UI.
Parameter | Type | Description |
---|---|---|
observedElement | HTMLElement | Required. Element to observe for color mode change. Example: document.body . |
isDarkModeCallback | (observedElement: Node) => boolean | Required. Function that returns true if dark mode otherwise false. Example: (observedElement) => observedElement.dataset.theme === 'dark' . |
colorModeAttribute | string | Required. Attribute that changes on the observedElement when colorMode changes. Example: "class" or "data-theme" . |
InkeepEmbeddedChatProps
This type represents the configuration for the Inkeep embedded chat widget.
Property | Type | Description |
---|---|---|
shouldAutoFocusInput | boolean | Determines whether to autofocus the chat input on load (only pertains to embedded chat). Default true . |
baseSettings | InkeepWidgetBaseSettings | Required. Base settings for any Inkeep widget. See reference here. |
aiChatSettings | InkeepAIChatSettings | AI chat settings for the Inkeep widget. See reference here. |
Multiple widgets
You may want to add mutliple Inkeep widgets on your site, like both a search bar and a chat button. In these cases, it can be helpful to create a common Inkeep JavaScript object that shares the same base settings for every widget.
const inkeepBase = Inkeep({
integrationId: envConfig.INTEGRATION_ID || "", // required
apiKey: envConfig.API_KEY || "", // required
organizationId: envConfig.ORGANIZATION_ID || "", // required
organizationDisplayName: "Inkeep",
primaryBrandColor: "black",
userId: "", // if you'd like analytics by user ID, like in cases where the user is authenticated or you have your own analytics platform
userEmail: "dev@inkeep.com",
userName: "Inkeep",
optOutAllAnalytics: false,
optOutAnalyticalCookies: false,
optOutFunctionalCookies: false,
});
You can then use inkeepBase.embed()
to add different components with the same base settings.
Changing props after initial render
Sometimes you may need to manage change settings after a widget has already been initialized, for example, to update user privacy preferences. To do this, you can use the render
method.
The below example illustrates how you change the primary color on the widget when a button is clicked.
const colors = [
'#26D6FF',
'#e300bd',
'#512fc9',
'#fde046',
'#2ecc71',
'#e74c3c',
'#9b59b6',
'#f1c40f',
];
let count = 0;
const changeColorButton = document.getElementById('change-color-button');
changeColorButton.addEventListener('click', () => {
count++;
inkeepWidget.render({
baseSettings: {
primaryBrandColor: colors[count % colors.length],
},
});
});