Style the components
Customize the search and chat widgets to match your brand.
The theme
object
The theme
object is part of the baseSettings
and can be used to customize the widgets to fit your brand’s style. It allows you to overrides variables that we use throughout our styling.
Fonts
Specify which fonts should be applied to the body, headings, or code (mono) elements. For example:
//...
baseSettings: {
//...
theme: {
tokens: {
fonts: {
heading: "'Space Grotesk'",
body: "'Inter'",
mono: "'Space Mono', monospace",
},
},
}
}
Colors
The widget ships with a neutral gray scheme and derives accent colors from the primaryBrandColor
(passed into baseSettings
), but these colors can be overridden with your own colors if you wish. Here is an example:
//...
baseSettings: {
//...
theme: {
tokens: {
colors: {
// grays for light mode
'gray.50': '#f8f9fa',
'gray.100': '#f1f3f5',
'gray.200': '#eceef0',
'gray.300': '#e6e8eb',
'gray.400': '#dfe3e6',
'gray.500': '#BDC2C7',
'gray.600': '#889096',
'gray.700': '#687076',
'gray.800': '#36424A',
'gray.900': '#11181c',
// grays for dark mode
'grayDark.50': '#ededed',
'grayDark.100': '#a0a0a0',
'grayDark.200': '#7e7e7e',
'grayDark.300': '#707070',
'grayDark.400': '#505050',
'grayDark.500': '#3e3e3e',
'grayDark.600': '#343434',
'grayDark.700': '#2e2e2e',
'grayDark.800': '#282828',
'grayDark.900': '#1c1c1c',
},
},
primaryColors: { // by default, derived from primaryBrandColor
textColorOnPrimary: '#11181c',
textBold: '#141d20',
textSubtle: '#354a51',
lighter: '#e5feff',
light: '#85f0ff',
lightSubtle: '#f1f8fa',
medium: '#26d6ff', // primaryBrandColor
strong: '#00b5dd',
stronger: '#006881',
hitContentPreview: '#00b5dd',
hitContentPreviewHover: '#006881',
textColorOnPrimary: 'white',
},
}
}
Z-indices
Customizing the zIndex tokens is usually not necessary but can be helpful if there is an element on your site with a high z-index that is being rendered on top of one of the widgets or modal. Below are the default token values. It is a good idea to update the rest of z-index tokens relative to the one you need to change, for example if changing modal
to 2000
make sure to also bump popover
to 2100
, skipLink
to 2200
etc.
//...
baseSettings: {
//...
theme: {
tokens: {
zIndex: {
hide: -1,
auto: 'auto',
base: 0,
docked: 10,
dropdown: 1000,
sticky: 1100,
banner: 1200,
overlay: 1300,
modal: 1400,
popover: 1500,
skipLink: 1600,
toast: 1700,
tooltip: 1800,
},
}
}
}
Search Bar
The Search Bar component has two variants to chose from subtle
and emphasized
, the default is subtle
. There are also four size variants to chose from expand
, compact
, shrink
, or medium
, the default is compact
. The Docusaurus package defaults to shrink
.

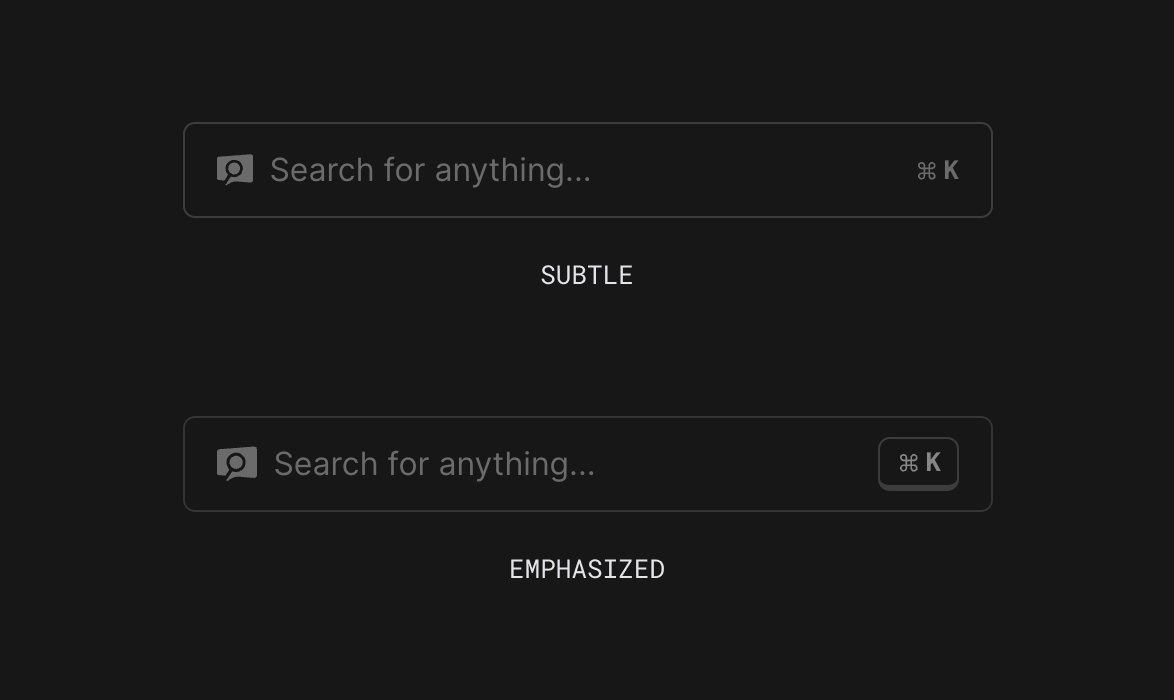
To change the variant and size:
//...
baseSettings: {
//...
theme: {
components: {
SearchBarTrigger: {
defaultProps: {
size: 'expand', // 'expand' 'compact' 'shrink' 'medium'
variant: 'emphasized', // 'emphasized' 'subtle'
},
},
}
}
}
Embedded Chat
Theme variants
The Embedded Chat component has two styling variants: container-with-shadow
and no-shadow
, the default is container-with-shadow
. There are also four size variants: shrink-vertically
, expand
, default
, or full-viewport
, the default is default
.
To change the variant and size:
//...
baseSettings: {
//...
theme: {
components: {
AIChatPageWrapper: {
defaultProps: {
size: 'shrink-vertically', // 'shrink-vertically' 'expand', 'default', 'full-viewport'
variant: 'no-shadow', // 'no-shadow' or 'container-with-shadow'
},
},
}
}
}
Centering and sizing
The embedded chat is sized relative to it’s container. By default, it expands to a certain max width and heights that preserve a reasonable aspect ratio for different breakpoints and tries to center itself relative to its parent. So it’s properly centered, consider wrapping it in parents that have the below stylings.
With a display='flex'
parent
With a display='block'
parent
Customizing dimensions (using size='expand'
)
We recommend setting the height for the container div to height: min(80vh, 800px);
Syntax highlighting theme
By default, the Prism syntax highlighting theme we use is oneLight in light mode and vsDark in dark mode. To use a different theme, you can provide it in the theme.syntaxHighlighter
prop.
For example, to use the dracula
theme:
import { themes } from "prism-react-renderer";
const inkeepProps = {
theme: {
syntaxHighlighter: {
lightTheme: themes.dracula, // theme used in light mode
darkTheme: themes.dracula, // theme used in dark mode
},
},
};
You can use published Prism themes or create your own.
Dark mode
Controlling the color mode
By default, the widgets will be rendered in light mode, to change the color mode use the forcedColorMode
prop in the colorMode
object of the baseSettings
.
//..
baseSettings: {
// ...
colorMode: {
forcedColorMode: 'dark', // options: 'light' or dark'
}
}
System color mode
To use the user’s system preference color mode, set the enableSystem
prop to true
(by default it is false
) in the colorMode
object of baseSettings
.
baseSettings: {
//...
colorMode: {
enableSystem: true,
}
}
Customize the icons
Bot avatar
The bot avatar can be customized by passing the botAvatarSrcUrl
and / or botAvatarDarkSrcUrl
(avatar to be used in dark mode) property to the aiChatSettings
. If botAvatarDarkSrcUrl
is not provided botAvatarSrcUrl
will be used in dark mode as well.
User avatar
The user avatar can be customized by passing the userAvatarSrcUrl
to the aiChatSettings
.
import { type InkeepAIChatSettings } from "@inkeep/uikit";
export const aiChatSettings: InkeepAIChatSettings = {
botAvatarSrcUrl: "http://example.com/bot-avatar.png",
botAvatarDarkSrcUrl: "http://example.com/bot-avatar-dark-mode.png",
userAvatarSrcUrl: "http://example.com/user-avatar.png",
// ...rest of aiChatSettings
};
Custom icons
These icons can be used by passing a dictionary called customIcons
in the baseSettings
. See example.
Property | Type | Description |
---|---|---|
search | CustomIcon | Search icon in search bar. |
thumbsUp | CustomIcon | Thumbs up icon. |
thumbsDown | CustomIcon | Thumbs down icon. |
messageCopy | CustomIcon | Copy message icon. |
codeCopy | CustomIcon | Copy code icon in code header. |
openLinkInNewTab | CustomIcon | Used on hover in chat citations and search results if shouldOpenLinksInNewTab is true. |
openLinkInSameTab | CustomIcon | Used on hover in chat citations and search results if shouldOpenLinksInNewTab is false. |
breadcrumbSeparator | CustomIcon | Breadcrumb separator icon. |
switchToSearch | CustomIcon | Switch to search icon. |
switchToChat | CustomIcon | Switch to chat icon. |
chatSubmit | CustomIcon | Chat submit icon. |
close | CustomIcon | Close icon. |
info | CustomIcon | Info icon next to the disclaimer and chat mode toggle. |
command | CustomIcon | Command icon in search bar. |
CustomIcon
Can either be builtIn
or custom
Property | Type | Description |
---|---|---|
builtIn | AvailableBuiltInIcons | Pass in the name of an icon that is already included in the widgets package. |
custom | string | URL of icon image. |
If the url for a custom
icon is a .svg
file it will be rendered as an <svg>
, all other file types will be rendered using an <img>
tag.
Custom icons example
import { type InkeepBaseSettings } from "@inkeep/uikit";
export const baseSettings: InkeepBaseSettings = {
customIcons: {
search: { builtIn: "IoSearch" }, // ex. using builtIn
thumbsUp: { custom: "/path/to/thumbs-up.svg" }, // ex. using custom
thumbsDown: { custom: "/path/to/thumbs-down.svg" },
messageCopy: { custom: "/path/to/message-copy.svg" },
codeCopy: { custom: "/path/to/code-copy.svg" },
openLinkInNewTab: { custom: "path/to/open-link-in-new-tab.svg" },
openLinkInSameTab: { custom: "path/to/open-link-in-same-tab.svg" },
breadcrumbSeparator: { custom: "path/to/breadcrumb-separator.svg" },
switchToSearch: { custom: "/path/to/switch-to-search.svg" },
switchToChat: { custom: "/path/to/switch-to-chat.svg" },
chatSubmit: { custom: "/path/to/chat-submit.svg" },
close: { custom: "/path/to/close.svg" },
info: { custom: "/path/to/info.svg" },
command: { custom: "/path/to/command.svg" },
},
// ...rest of baseSettings
};
Custom CSS and styling
Additional customization can be done via CSS stylesheets. Because the Inkeep widgets are isolated via a shadow DOM, any custom styling must be passed to the widget configuration in order for them to be applied.
InkeepStyleSettings
Property | Type | Description |
---|---|---|
stylesheetUrls | ReactElement[] | Array of urls to stylesheets with style overrides. |
stylesheets | string[] | Array of <link> or <style> tags with style overrides. |
Via stylesheetUrls
Pass the url(s) of the stylesheet(s):
const inkeepEmbeddedChatProps: InkeepEmbeddedChatProps = {
baseSettings: {
// ... typeof InkeepBaseSettings
theme: {
stylesheetUrls: ["cdn.com/path-to-stylesheet.css"],
},
},
//...
};
The urls can be relative or absolute.
Via stylesheets
Alternatively if using React, you may instead pass an array of <link>
or <style>
tags to the stylesheets
property. Please ensure that each element has a unique key. For example:
const inkeepEmbeddedChatProps: InkeepEmbeddedChatProps = {
baseSettings: {
// ... typeof InkeepBaseSettings
theme: {
stylesheets: [
<link
key="my-stylesheet-key"
rel="stylesheet"
href="cdn.com/path-to-stylesheet.css"
/>,
],
},
},
//...
};
Hosting the stylesheet
Please ensure that stylesheets are in valid, publicly accessible URLs and correctly load. If a stylesheet does not load, the Inkeep widgets will not render.
You can host your stylesheet in a CDN or include it as part of the public assets exposed in an existing application.
For example:
If using Next.js, you can create a css file within the public folder and then pass that url to the stylesheetUrls
prop. For example:
// for this example there is a file in the public folder is called inkeep.css
const inkeepEmbeddedChatProps: InkeepEmbeddedChatProps = {
baseSettings: {
// ... typeof InkeepBaseSettings
theme: {
stylesheetUrls: ["/inkeep.css"],
},
},
//...
};
Class names
Below is a list of class names and their corresponding component part:
Main widget containers
className | Element |
---|---|
.ikp-ai-chat-page-wrapper | EmbeddedChat |
.ikp-search-bar-trigger__container | SearchBar |
.ikp-floating-button | ChatButton |
.ikp-modal-widget__container | Modal |
Search
className | Element |
---|---|
.ikp-search-bar-trigger__wrapper | search bar trigger |
.ikp-search-bar-trigger__container | search bar trigger |
.ikp-search-bar-trigger__icon | search bar trigger |
.ikp-search-bar-trigger__search-icon | search bar trigger |
.ikp-search-bar-trigger__kbd | search bar trigger |
.ikp-kbd-shortcut-icon | search bar trigger |
.ikp-search-bar-stand-alone__search-icon | search bar modal |
.ikp-search-bar-stand-alone__search-input | search bar modal |
.ikp-search-bar-stand-alone | search bar modal |
.ikp-ask-ai-card | search bar modal |
.ikp-switch-to-chat-icon | search bar modal |
.ikp-search-result-tabs | search results |
.ikp-search-result-tabs__list | search results |
.ikp-search-result-tab | search results |
.ikp-search-result-tab__content | search results |
.ikp-search-result-content__breadcrumbs | search results |
.ikp-search-result-content__header | search results |
.ikp-preview-content | search results |
.ikp-preview-content__highlight | search results |
.ikp-search-result-line__first | search results |
.ikp-search-result-line__second | search results |
.ikp-result-card__icon | search results |
.ikp-open-link-in-new-tab-icon | search results |
.ikp-open-link-in-same-tab-icon | search results |
.ikp-tagline | search footer |
AI chat
className | Element |
---|---|
.ikp-ai-chat-page-wrapper | ai chat |
.ikp-ai-chat__scrollable-page-wrapper | ai chat |
.ikp-ai-chat__header | ai chat |
.ikp-ai-chat__header-toolbar | ai chat |
.ikp-ai-chat__scrollable-page-content | ai chat |
.ikp-ai-chat__scrollable-page-content__inner | ai chat |
.ikp-messages__wrapper | messages |
.ikp-messages__error | messages |
.ikp-message__wrapper | message |
.ikp-message__divider-wrapper | message |
.ikp-message__divider | message |
.ikp-chat-bubble__wrapper | chat bubble |
.ikp-chat-bubble__user-message | chat bubble (user) |
.ikp-chat-bubble__bot-message | chat bubble (bot) |
.ikp-chat-bubble__message-content-wrapper | chat bubble |
.ikp-chat-bubble__user-message-content-wrapper | chat bubble |
.ikp-chat-bubble__user-message-content | chat bubble |
.ikp-chat-bubble__message-actions | chat bubble |
.ikp-chat-bubble__message-actions-copy | chat bubble |
.ikp-thumbs-up-down__wrapper | thumbs up and down |
.ikp-thumbs-up__button | thumbs up button |
.ikp-thumbs-up-icon | thumbs up icon |
.ikp-thumbs-down__button | thumbs down button |
.ikp-thumbs-down-icon | thumbs down icon |
.ikp-message-header | ai chat |
.ikp-message-header__avatar-box | ai chat |
.ikp-bot-avatar__box | ai chat |
.ikp-quick-questions__label | quick questions |
.ikp-quick-question-button__wrapper | quick questions |
.ikp-quick-question-button__highlight | quick questions |
.ikp-quick-question-button | quick questions |
.ikp-ai-chat__disclaimer | disclaimer |
.ikp-ai-chat__disclaimer-label | disclaimer |
.ikp-disclaimer-icon | disclaimer |
.ikp-ai-chat-footer__content-wrapper | chat footer |
.ikp-ai-chat-footer__content | chat footer |
.ikp-ai-chat-footer | chat footer |
.ikp-ai-chat-footer__footer | chat footer |
.ikp-ai-chat__message-box-wrapper | message box |
.ikp-ai-chat__message-box | message box |
.ikp-ai-chat__message-box-row | message box |
.ikp-ai-chat__send-icon | submit chat icon |
.ikp-ai-chat__message-input | message input |
.ikp-ai-chat__send-button | submit chat button |
.ikp-tagline | chat footer |
.ikp-get-help__button | get help |
.ikp-get-help__menu | get help |
.ikp-get-help__menu-item | get help |
.ikp-get-help__menu-item-link | get help |
.ikp-ai-chat__toolbar | ai chat modal |
.ikp-ai-chat__toolbar-header | ai chat modal |
.ikp-switch-to-search-button | ai chat modal |
.ikp-switch-to-search-icon | ai chat modal |
.ikp-citation-sources-header | ai chat |
.ikp-result-card | result card |
.ikp-result-card--focused | result card (focused state) |
.ikp-result-card__container | result card |
.ikp-result-card__container--focused | result card (focused state) |
.ikp-result-card__body | result card |
.ikp-result-card__content | result card |
.ikp-result-card__content-text | result card |
.ikp-result-card__icon | ai chat |
.ikp-result-card__hover-icon | ai chat |
.ikp-search-result-line__first | result card |
.ikp-search-result-line__second | result card |
.ikp-bread-crumb-separator | result card |
.ikp-codeblock-container | code block |
.ikp-codeblock-header | code block |
.ikp-codeblock-header__language-wrapper | code block |
.ikp-codeblock-header__language | code block |
.ikp-codeblock-header__copy-button-wrapper | code block |
.ikp-codeblock-header__copy-button | code block |
.ikp-copy-code-icon | code block |
.ikp-codeblock-highlighter-wrapper | code block |
.ikp-codeblock-highlighter | code block |
.ikp-content-parser__sup | message content |
.ikp-content-parser__sup-link | message content |
.ikp-content-parser__text | message content |
.ikp-content-parser__ol | message content |
.ikp-content-parser__li | message content |
.ikp-content-parser__code | message content |
.ikp-loading__dots | ai chat |
.ikp-loading__dot | ai chat |
.ikp-loading__status-text | ai chat |
Chat button
className | Element |
---|---|
.ikp-floating-button | chat button |
.ikp-floating-button-box | chat button |
.ikp-chat-button__text | chat button |
.ikp-bot-avatar__box | chat button |
Modal
className | Element |
---|---|
.ikp-modal-widget__container | modal container |
.ikp-modal-widget__content | modal content |
.ikp-modal__backdrop | modal overlay |
.ikp-switch-view-toggle | modal view toggle wrapper |
.ikp-switch-view-toggle__button | modal view toggle button |
.ikp-switch-view-toggle__button--active | modal view toggle button (active) |
Using Inkeep CSS variables
Inkeep CSS variables can be utilized within stylesheets to customize elements. For example, the Chat Button uses a dark gray color by default. You can use the .ikp-floating-button
and any of the pre-defined theme color tokens or any color of your choice to customize it:
.ikp-floating-button {
background: var(--ikp-colors-inkeep-primary-medium);
}
Dark mode style overrides
To apply a specific style for dark mode only, utilize the [data-theme='dark']
attribute. For example:
[data-theme="dark"] .ikp-floating-button {
background: #353e52;
}