Ui componentsTools
Escalate to Support
Dynamically direct users to support as needed
Overview
You can use tools to identify when the AI assistant can't fully address a user's question and provide appropriate support options.
A button can be shown in the assistant message that can use actions to:
- Link to a support page
- Have the user fill out a support form
- Transfer to live chat
Note
Tools are available for the Enterprise tier. Please contact sales@inkeep.com for details.
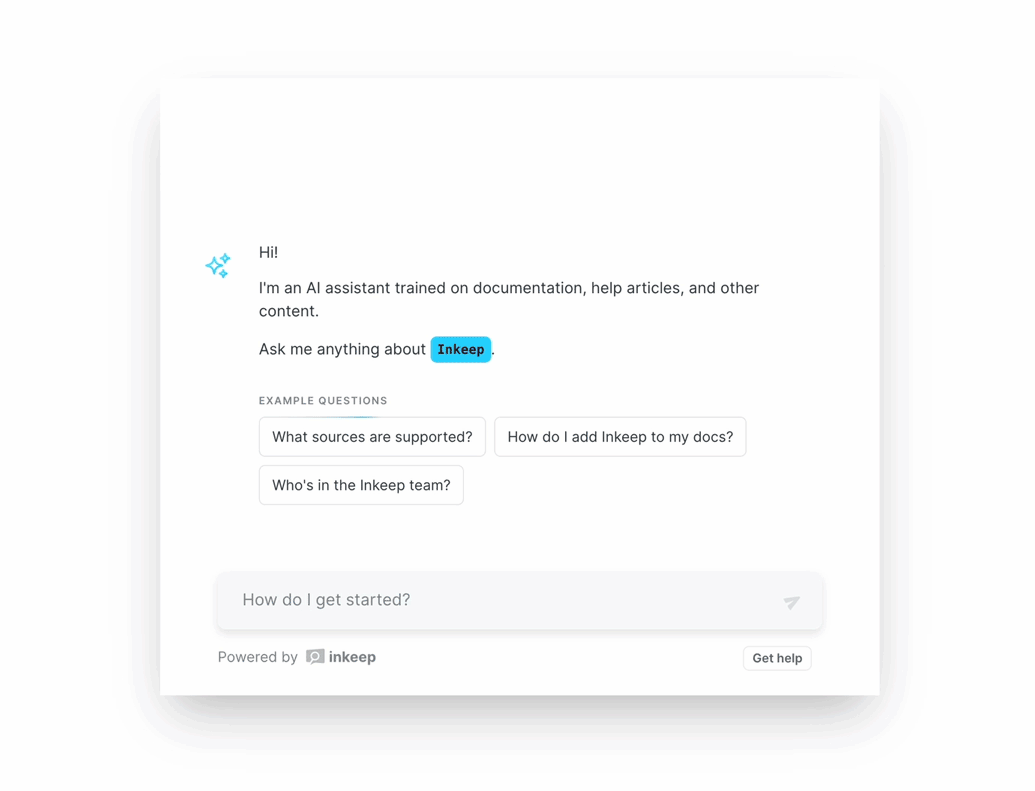
Answer Confidence Tool
This example uses the provideAnswerConfidenceSchema
from Common Tools to determine the AI assistant's confidence level. You can define your own classifications or logic for the escelation paths you prefer.
When the answer is not confident, we can show a dynamic button to escalate to support.
Link to Support Page
Support Ticket Form
This example embeds a form for creating support tickets directly within the chat interface: