Ui componentsTools
Detect Sales Signals
Copy page
Identify and respond to purchasing signals in user questions
Overview
The "Detect Sales Signals" tool pattern helps identify when users express potential interest in making a purchasing or action related to sales. This is useful for embedding call to actions to:
- Schedule a sales meeting
- Triggering in-app logic to make an upgrade
- Guiding users to relevant pricing or sales content
Note
Tools are available for the Enterprise tier. Please contact sales@inkeep.com for details.
Book a meeting
This example provides a demo scheduling link when a sales signal is detected.
Use the following styles if you would like to make the button larger and on it's own line:
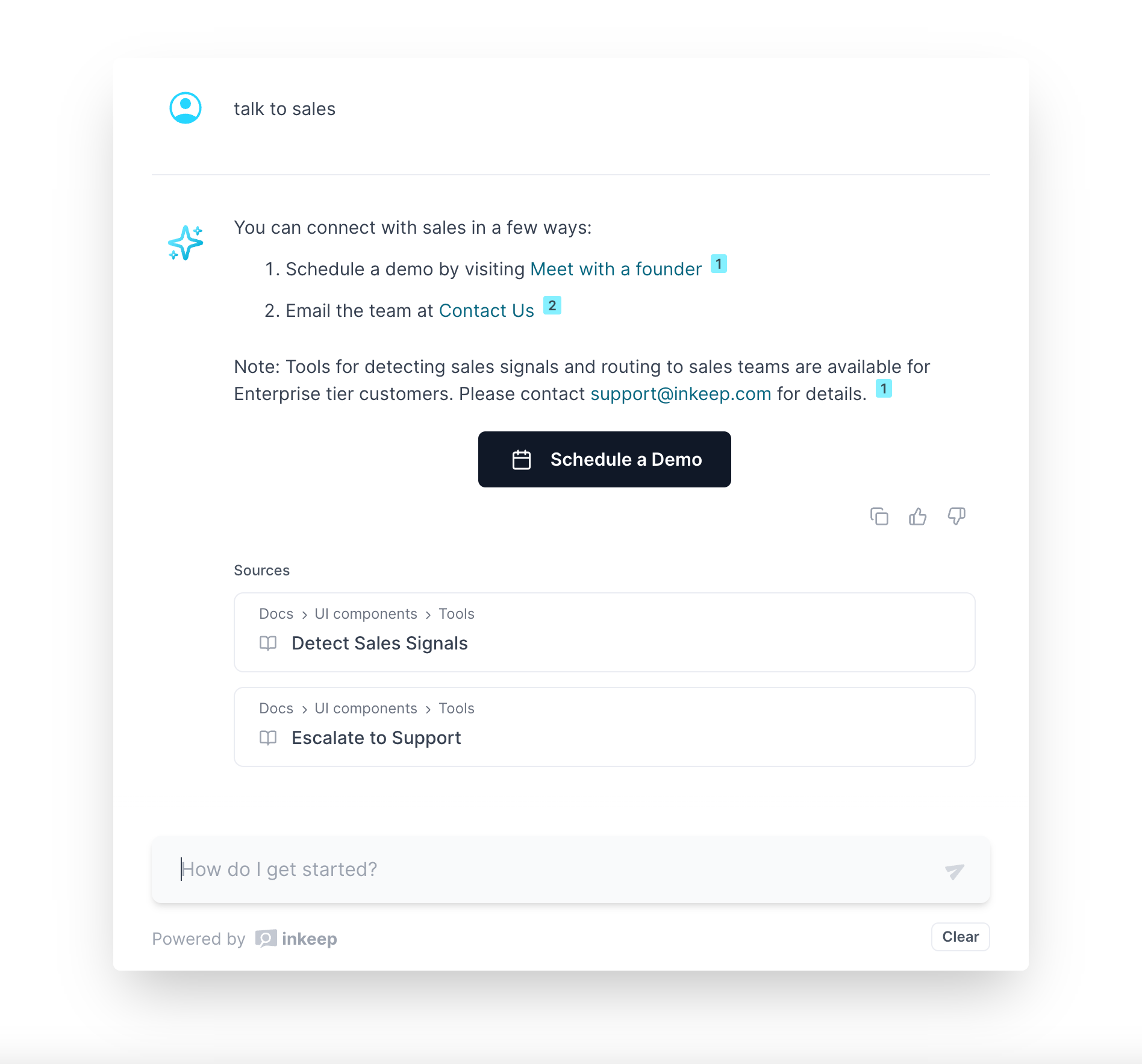
Signal-Based Actions
This example provides different actions based on the specific type of sales signal detected.
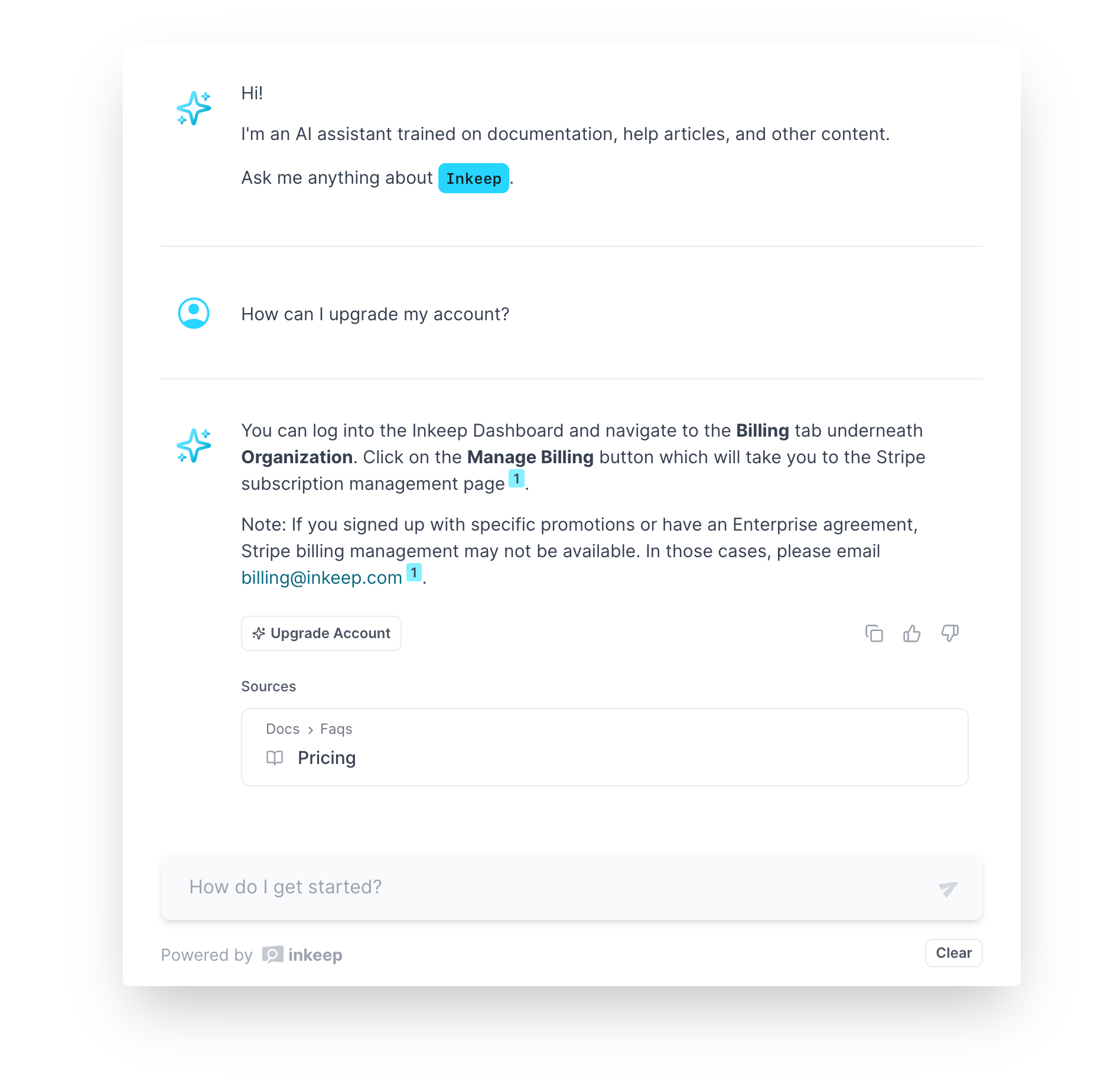