Ui componentsCommon settings
Actions
Copy page
Use actions to add custom behavior to the UI Components.
Actions allows you to define interactive flows in your chat interface, such as opening a link, opening an embeded form, or executing custom JavaScript. These actions can be triggered by users, when they click on buttons, or the AI assistant.
Action Types
Open URL Action
Opens a URL in a new tab or the current window:
Invoke Callback Action
Executes a callback function with optional modal control:
Open Form Action
Opens a form dialog with configurable fields and success view:
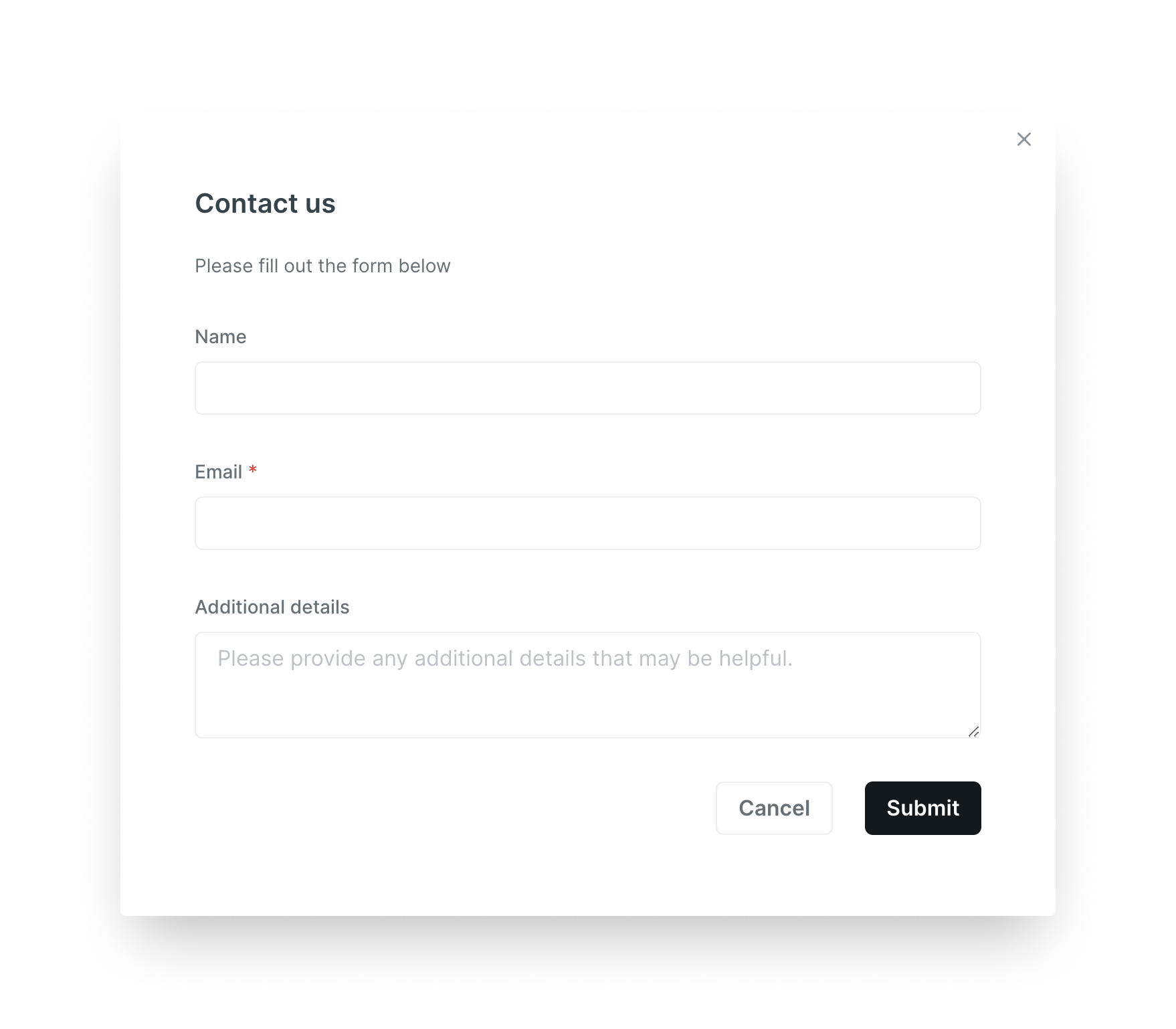
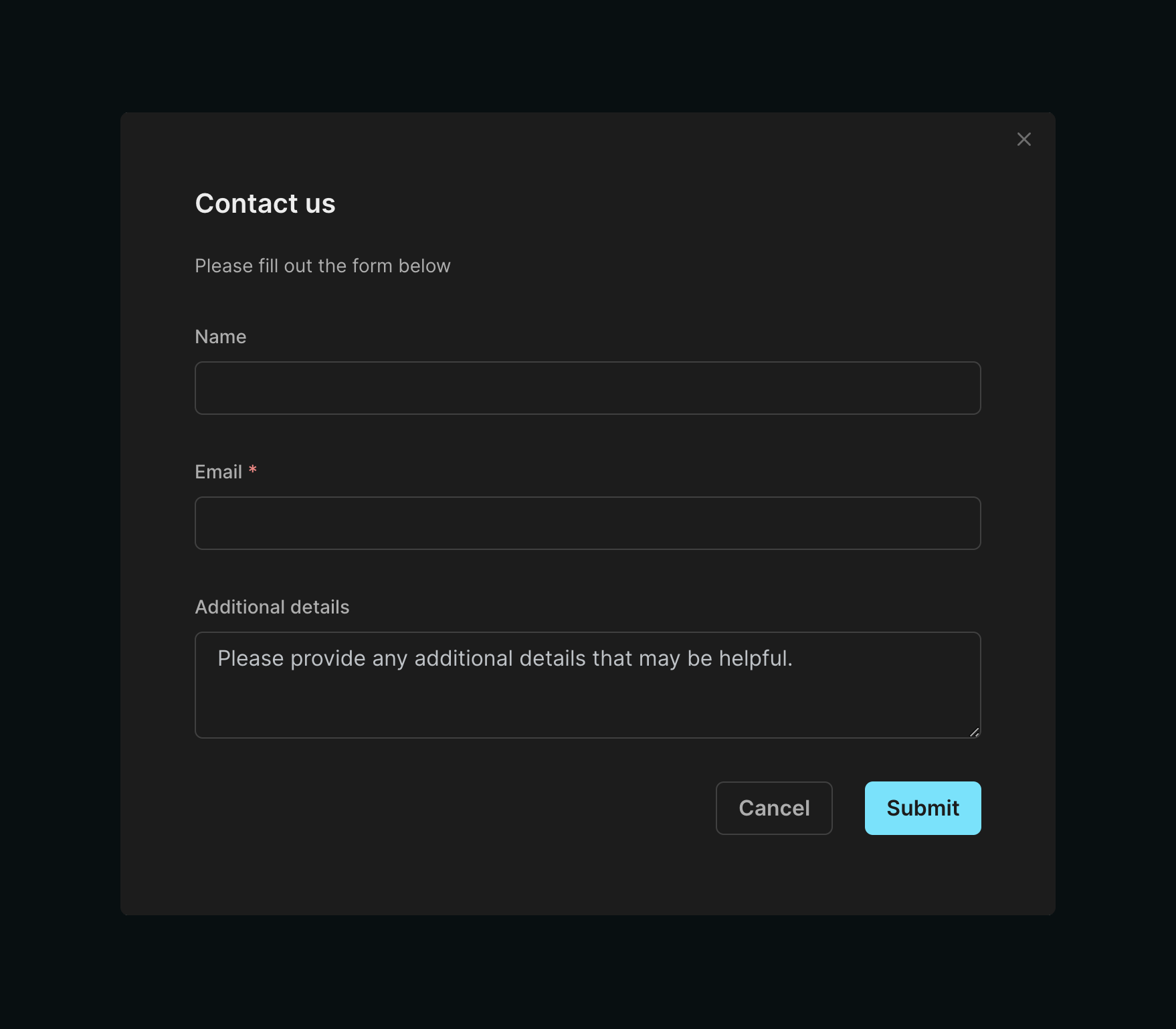
See Form Settings for a full reference on what can be configured in the form.
Support Action Examples
Below are some examples of common actions used to hand off to your support channels.