React
Embedded Chat (React)
Add a chat UI directly on a dedicated React powered page.
Overview
An "embedded chat" is a chat UI that is directly incorporated into a page instead of within a pop-up modal. The embedded chat UI looks like this:
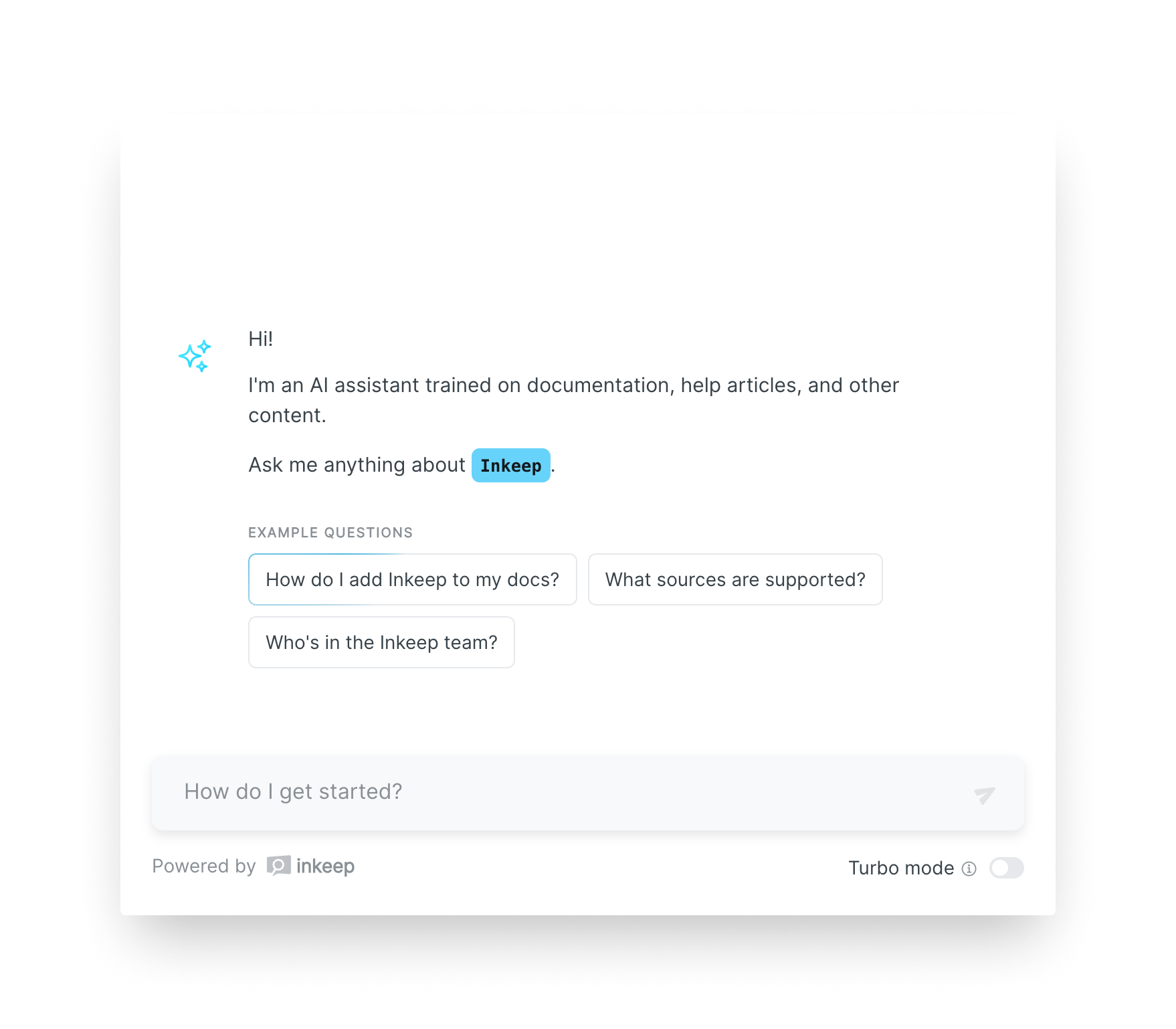
Scenarios
You may want to add an embedded chat in places where you want to encourage the user to interact with the AI chat directly. For example, an embedded chat can be useful to:
- Deflect questions in your help center site
- Provide an AI chat experience if your documentation site doesn't allow third party components, like for GitBook and Readme.
- Create a place for sharable chat sessions
You can incorporate the embedded chat directly on an existing page like help.domain.com
or create a dedicated one like domain.com/ask-ai
.
Quick Start
Install the component library
npm install @inkeep/uikit
Define the component
import {
InkeepEmbeddedChat,
type InkeepEmbeddedChatProps,
} from "@inkeep/uikit";
const baseSettings: InkeepBaseSettings = {
apiKey: process.env.INKEEP_API_KEY!,
integrationId: process.env.INKEEP_INTEGRATION_ID!,
organizationId: process.env.INKEEP_ORGANIZATION_ID!,
organizationDisplayName: "Inkeep",
primaryBrandColor: "#26D6FF",
};
const inkeepEmbeddedChatProps: InkeepEmbeddedChatProps = {
baseSettings: {
...baseSettings,
},
aiChatSettings: {
// ... typeof InkeepAIChatSettings
botAvatarSrcUrl: "/img/inkeep-logo.svg", // use your own bot avatar
botAvatarDarkSrcUrl: "/img/inkeep-logo-dark.svg" // for dark mode or more contrast against button bg
quickQuestions: [
"Example question 1?",
"Example question 2?",
"Example question 3?",
],
},
};
export const EmbeddedChat = () => {
return (
<div>
<InkeepEmbeddedChat {...inkeepEmbeddedChatProps} />
</div>
);
};
InkeepEmbeddedChatProps
This type represents the configuration for the Inkeep embedded chat widget.
Property | Type | Description |
---|---|---|
shouldAutoFocusInput | boolean | Determines whether to autofocus the chat input on load (only pertains to embedded chat). Default true . |
baseSettings | InkeepBaseSettings | Required. Base settings for any Inkeep widget. See reference here. |
aiChatSettings | InkeepAIChatSettings | AI chat settings for the Inkeep widget. See reference here. |
Example
import type { InkeepEmbeddedChatProps } from "@inkeep/uikit";
import baseSettings from "./baseSettings"; // your base settings typeof InkeepBaseSettings
const inkeepEmbeddedChatProps: InkeepEmbeddedChatProps = {
shouldAutoFocusInput: true,
baseSettings: {
...baseSettings,
},
aiChatSettings: {
//... typeof InkeepAIChatSettings
},
};